Online tech forums can feel intimidating. Three simple things can greatly improve your experience & odds for useful help, regardless of your skill level.
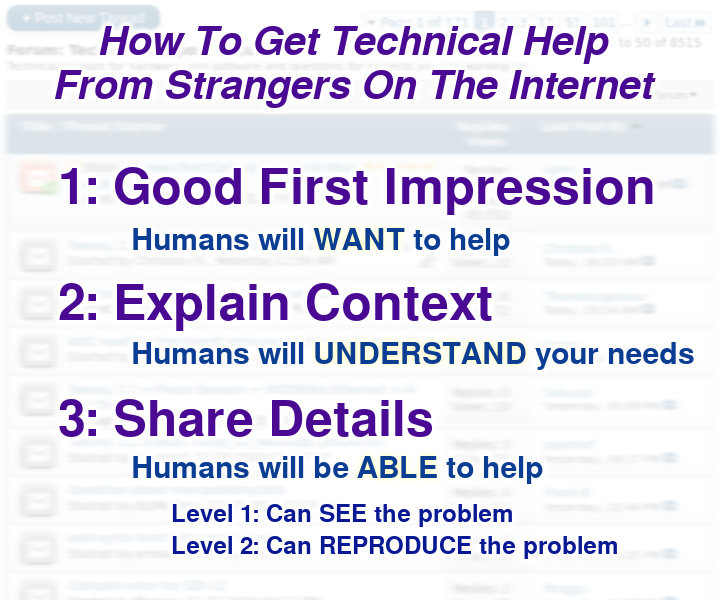
Good First Impression
“You get 1 chance to make a good first impression” is timeless wisdom. Strangers will quickly form an opinion of you, based only on the words, images or video in your message. Make your words count!
Showing you’ve made an effort, more than any other factor, will influence people to help.
But if you’re an absolute beginner, what sort of effort can reasonably be expected? Anyone can try a Google search using the words from their question. Many experts have long forgotten how difficult finding relevant info can be when you don’t know the proper terms, or unrelated tech has since started using those words. Just explaining the search you tried and confusing or off-topic info you’ve seen can go a long way towards helping seasoned experts to understand your struggle. The results aren’t important, your personal effort is what matters!
Genuine interest to learn also tends to make a good impression. Even a few words can really communicate your attitude. Frustrated but determined to learn from a trying experience can make a great impression. Very smart people who can help the most tend to appreciate genuine & candid communication.
Of course if you have started a project or done work, mention it. Or better, show what you have tried. Screenshots, photos or even a quick video can powerfully demonstrate what you’ve done, and cover the other 2 aspects of a successful forum question.
Even just a few extra words, which show you are making a sincere effort and sincerely wish to learn, can make an improvement in the response you’ll likely receive.
Context Brings Understanding
Humans have amazing capability to genuinely understand. Experts can sometimes apply their tremendous knowledge towards creative ideas to solve your problem… when they actually understand what you really need.
Providing context in your question is key. What are your larger goals? How does the question you’re asking fit into your project? What do you ideally hope to accomplish? And why?
When working on a technical matter, it’s easy to focus on only the issue at hand. Remember, good people with vast knowledge & experience regularly read forums, just because they enjoy helping others. When composing your forum question, don’t forget to give context. It can make the difference between answers that are at best technically correct and answers that are truly insightful.
Details Are Essential
Modern technical issues can involve a mind boggling number of details. How much raw information should your question include? Before posting, try asking these questions:
- Can readers SEE or EXPERIENCE your problem?
- Can readers REPRODUCE your problem?
It’s easy to joke “I saw an error, but clicked OK without reading”. But seriously, a basic level of detail allows everyone reading your message to see the problem. A screenshot or exact copy of an error, plus specific information about software, hardware, and steps taken are a baseline for your readers to merely attempt to see the problem as you did.
Ideally, people reading your message would be able to reproduce the problem. Providing this level of detail is not always practical. But if you can give enough info, odds of someone solving the problem are vastly improved.
Software programming questions should include complete source code, not merely the small fragments. Often code issues involve subtle details, such as declarations of variables elsewhere in the code. Some experts can spot these sorts of problems with amazing speed, if you show complete code! Don’t forget to be specific about any extra libraries used.
Electronic hardware issues usually need photos or accurate wiring diagrams, and links or part numbers of materials used.
It’s common to feel nervous or self-conscious about showing your project details on a forum. Don’t be shy. Experts who regularly read the forum genuinely want to help. Show enough detail, give context and demonstrate you’ve made some effort and odds very good they will help!
Common Pitfalls
Forums don’t always work ideally. Usually the 3 steps above will lead to good results, but there are a number of common problems to keep in mind.
Too Much Diagnosis
Technical problem solving involves observing the effects of an issue and trying to deduce the cause. A very natural human tendency writing while in this mindset is to focus moreso on the presumed causes than objectively sharing the observed effects.
Usually a short break between an intense debugging session and composing your forum post can make a tremendous difference. When writing, try to think of your reader’s perspective. Are you asking people to help diagnose the problem, or merely asking them to nod “yup, you’re right” in agreement with your existing conclusions?
However, sharing your thought process can avoid people needlessly covering the same ground you’ve already investigated. There is no one perfect way. Just keep in mind that too much diagnosis can shut people out of helping you troubleshoot.
Venting Frustration
Let’s face it, some technical problems are really hard, even intensely frustrating. When you’re annoyed, venting frustration is a very natural human tendency.
Before you post on a forum, creating that all-important first impression with strangers, review your message. Some level of expressed frustration is normal and maybe even helpful. It can show you’ve really tried. Just beware the common trap, where your forum message ends up being perceived as a rant rather than a question or request for help.
Nobody Replies
Even under ideal conditions, sometimes forum posts get no replies. This can feel disappointing & disheartening. Try not to let that emotion take control as you compose a reply to “bump” the thread. You can do much better.
Effort matters. If days or weeks have elapsed since your first post, presumably you’ve put more effort into the project? Even if fruitless, the personal effort you’ve invested since your prior post is something you can share that tends to draw people in. Photos or code or other clear signs of work on your part can be highly effective.
Sometimes very difficult questions, with plenty of detail, get no replies simply because nobody knows the answer. Or nobody knows with certainty. Asking for opinions or ideas for directions to try can really help in these cases, turning what started as a solid question into a more fluid conversation. Again, apparent effort is the key factor that attracts people to contribute towards helping solve a tough problem.
Most forums discourage or even ban posting duplicate copies of your question, or “cross posting”. Posting the exact same message in multiple places at the same time is almost never a good idea. Experts who regularly read the forums, the people most likely to help you, will almost certainly notice. You get one chance to make a good first impression. However, after a period with no answers, sometimes duplicate posting can be done gracefully, usually with an explanation & link to the prior unanswered question.
Academically Dishonest Students
Every tech forum gets lazy students asking people to do their homework. Experts who regularly read forums are inundated by these low-quality questions. Like an email inbox with spam, the last thing you want is your message to be mistaken for more junk & quickly dismissed.
If you are doing a student project, usually the best approach is to be honest about the academic nature (and deadline) and to make sure your sincere effort shines through. Earnest effort and genuine desire to learn is what distinguishes good students in the minds of experts who will actually help!
Proprietary Projects
When you can’t share source code or other relevant technical details, technical forum help is rarely effective.
Usually you must invest extra effort to separate just the one piece that is problematic from the rest of your project, so it can be shared. If you have not done this, consider that asking “has anyone seen a problem like XYX” is essentially asking humans to function as a search engine. Sometimes the results are good (better than you could do with Google), but difficult technical problems without sharing relevant details are rarely solved by blind guessing.
If your employer or organization absolutely will not allow any code or details shared, no matter how trivial, perhaps using “enterprise” products which come with support contracts, or hiring a private consulting firm is more appropriate than asking on a public forum.
After Your Question Is Answered
The best way to say “Thank You” is to acknowledge who had the right answer. Many experts pour countless unpaid hours into helping strangers on forums, simply because it feels good to be helpful. Being recognized as supplying the correct answer is a nice reward.
When your problem is solved, please consider Google and other search engines may send people with similar technical questions to your messages for years to come. The very best thing you can do after a technical problem is resolved is a quick but clear message confirming the solution.
If you posted on multiple forums, please take a few minutes to follow up on every thread with a link to the message with best answer.
About This Article (and Me)
Over the last 6 years, I’ve written 18620 answers our forum here at PJRC, and many more on numerous other forums. During this time, we’ve managed to grow a pretty good forum community and help many thousands of people with projects.
I watched and tried to learn which what has worked and how we might improve. One thing that’s become very clear is so many people need a little guidance on how to best ask their questions. It’s not the technical nature, but these subtle human factors that matter most.
My hope is to see all tech forums improve. Please, share this article.
-Paul Stoffregen