Audio Connections & Memory
AudioConnection objects route the output of an output of any audio object to the input of another. Useful audio applications usually require several audio objects and of course connections between them.Audio data is stored in 128 sample blocks, which you allocate with AudioMemory. Functions described below allow you to monitor the audio library's usage of this memory.
Usage
AudioConnection myConnection(source, sourcePort, destination, destinationPort);Route audio data from the source object's sourcePort, to the destination object's destinationPort.
AudioConnection myConnection(source, destination);Route audio data from the source object's output 0, to the destination object's input 0. This shorter form is convenient when using objects with only a single input and output.
AudioConnection myConnection();Create a disconnected AudioConnection. It does not route any audio data until the connect() function is used.
myConnection.connect(source, sourcePort, destination, destinationPort);Cause a disconnected AudioConnection to begin routing audio data, from the source object's sourcePort, to the destination object's destinationPort.
myConnection.connect(source, destination);Cause a disconnected AudioConnection to begin routing audio data, from the source object's output 0, to the destination object's input 0.
myConnection.connect();Cause a disconnected AudioConnection to begin routing audio data, using previously configured settings.
myConnection.disconnect();Stop routing audio data. To reconfigure an AudioConnection, it must first be disconnected before calling connect() with different parameters.
AudioMemory(numberBlocks);Allocate the memory for all audio connections. The numberBlocks input specifies how much memory to reserve for audio data. Each block holds 128 audio samples, or approx 2.9 ms of sound. Usually an initial guess is made for numberBlocks and the actual usage is checked with AudioMemoryUsageMax().
AudioMemoryUsage();Returns the number of blocks currently in use.
AudioMemoryUsageMax();Return the maximum number of blocks that have ever been used. This is by far the most useful function for monitoring memory usage.
AudioMemoryUsageMaxReset();Reset the maximum reported by AudioMemoryUsageMax. If you wish to discover the worst case usage over a period of time, this may be used at the beginning and then the maximum can be read.
Connection Rules & Guidelines
Normally a single output connects to a single input.You may send the output from one object to as many inputs as you like. The audio library efficiently handles objects sharing the output of an object.
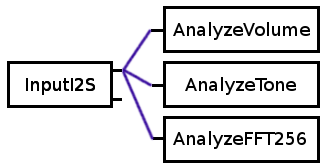
However, each input can receive only a single connection. If you attempt to connect many outputs to a single input, only the last connection will work.
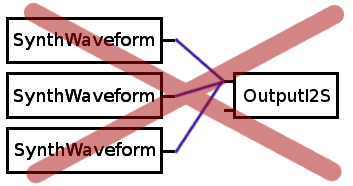
To feed multiple outputs to a single input, use the Mixer object to combine them to a single output.
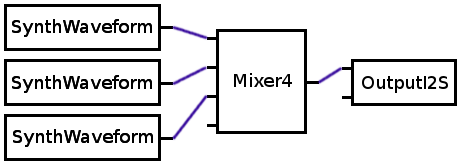
Audio objects should be created in the order data is processed, inputs, playback and synthesis, then effects, filters, mixers, and lastly outputs.
Connections are most efficient when made from an earlier object (in the order they are created) to a later one. Connections from a later object back to an earlier object can be made, but they add a 1-block delay and consume more memory to implement that delay.