TimeAlarms Library
TimeAlarms, by Michael Margolis, runs functions at specific times. It is means to be used together with the Time library
Download: |
Included with the Teensyduino Installer Latest Developments on TimeAlarms |
Hardware Requirements
TimeAlarms does not require any special hardware, because it uses the time and date provided by the Time library.Creating Alarms
Alarms are used to call a function at a specific time of the day.
Alarm.alarmRepeat(hours, minutes, seconds, function);Create an alarm that will call a function every day at a particular time.
Alarm.alarmRepeat(dayofweek, hours, minutes, seconds, function);
Create an alarm that will call a function every week on a specific day at a particular time.
"dayofweek" can be dowSunday, dowMonday, dowTuesday, dowWednesday,
dowThursday, dowFriday, or dowSaturday.
Create an alarm that will call a function tomorrow at a particular time.
Alarm.alarmOnce(dayofweek, hours, minutes, seconds, function);Create an alarm that will call a function once, at specific day and time.
Creating Timers
Timers call a function at regular intervals.
Alarm.timerRepeat(seconds, function);Create a timer that will call a function every at an interval of "seconds".
Alarm.timerOnce(seconds, function);Create a timer that will call a function once in "seconds".
Normal Running Usage
Alarm.delay(milliseconds);Alarms and Timers are only checks and their functions called when you use this delay function. You can pass 0 for minimal delay. This delay should be used instead of the normal Arduino delay(), for timely processing of alarms and timers.
Example Program
You can open this example from File > Examples > TimeAlarms > TimeAlarmExample.
/* * TimeAlarmExample.pde * * This example calls alarm functions at 8:30 am and at 5:45 pm (17:45) * and simulates turning lights on at night and off in the morning * A weekly timer is set for Saturdays at 8:30:30 * * A timer is called every 15 seconds * Another timer is called once only after 10 seconds * * At startup the time is set to Jan 1 2011 8:29 am */ #include <Time.h> #include <TimeAlarms.h> void setup() { Serial.begin(9600); setTime(8,29,0,1,1,11); // set time to Saturday 8:29:00am Jan 1 2011 // create the alarms Alarm.alarmRepeat(8,30,0, MorningAlarm); // 8:30am every day Alarm.alarmRepeat(17,45,0,EveningAlarm); // 5:45pm every day Alarm.alarmRepeat(dowSaturday,8,30,30,WeeklyAlarm); // 8:30:30 every Saturday Alarm.timerRepeat(15, Repeats); // timer for every 15 seconds Alarm.timerOnce(10, OnceOnly); // called once after 10 seconds } void loop(){ digitalClockDisplay(); Alarm.delay(1000); // wait one second between clock display } // functions to be called when an alarm triggers: void MorningAlarm(){ Serial.println("Alarm: - turn lights off"); } void EveningAlarm(){ Serial.println("Alarm: - turn lights on"); } void WeeklyAlarm(){ Serial.println("Alarm: - its Monday Morning"); } void ExplicitAlarm(){ Serial.println("Alarm: - this triggers only at the given date and time"); } void Repeats(){ Serial.println("15 second timer"); } void OnceOnly(){ Serial.println("This timer only triggers once"); } void digitalClockDisplay() { // digital clock display of the time Serial.print(hour()); printDigits(minute()); printDigits(second()); Serial.println(); } void printDigits(int digits) { Serial.print(":"); if(digits < 10) Serial.print('0'); Serial.print(digits); }
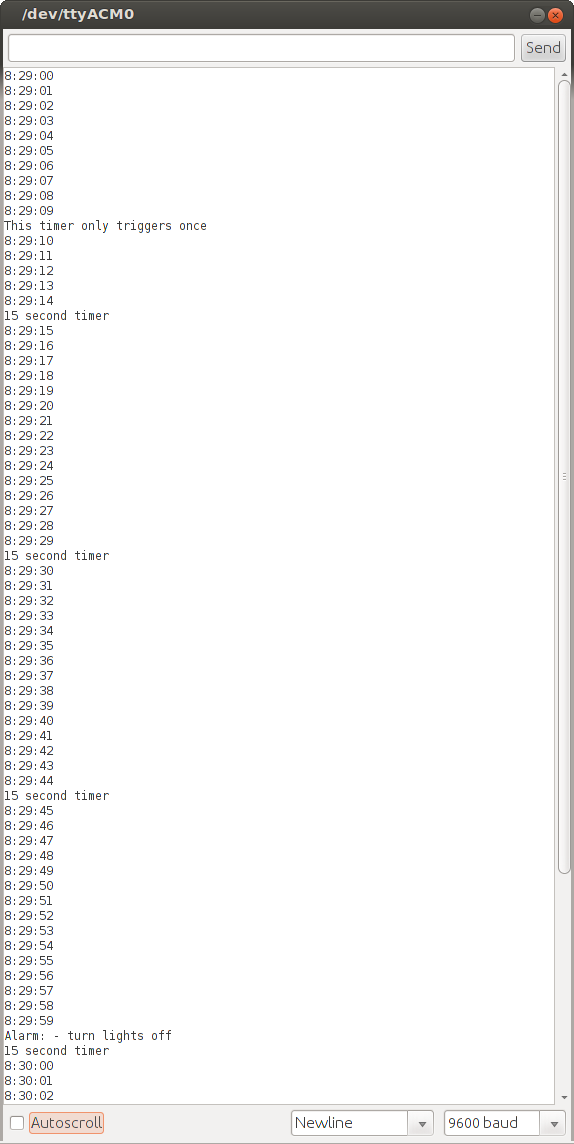