LedDisplay Library
LedDisplay lets you use a Avago HCMS-29xx type display. These are small, very bright and easily readable 4 or 8 character displays. They are also quite expensive.
Download: |
Included with the Teensyduino Installer Latest Developments on Github |
Hardware Requirements
You will need a Avago HCMS-29xx display. The "xx" numbers represent the size and color, but all of them work the same way. This photo shows a HCMS-2913.
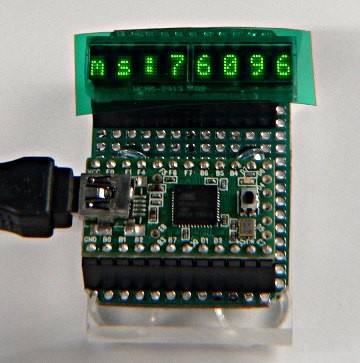
Basic Usage
LedDisplay myDisplay = LedDisplay(data, rs, clock, enable, reset, displayLength)Create a LedDisplay object which prints to your HCMS-29xx display. The first 5 numbers are the pins where you connected the signals, and the last is the number of characters (either 4 or 8). You can connect more than on HCMS-29xx and create a separate LedDisplay object to print to each.
myDisplay.begin()Initialize the HCMS-29xx display.
myDisplay.setBrightness(brightness)Set the brightness, between 0 to 15.
myDisplay.home()Set the printing position to the first (left most) character.
myDisplay.print(data)Print text or numbers to the display.
Example Program
File > Examples > LedDisplay > LedDisplay_print
#include <LedDisplay.h> // Define pins for the LED display. // You can change these, just re-wire your board: #define dataPin 6 // connects to the display's data in #define registerSelect 7 // the display's register select pin #define clockPin 8 // the display's clock pin #define enable 9 // the display's chip enable pin #define reset 10 // the display's reset pin #define displayLength 8 // number of characters in the display // create am instance of the LED display library: LedDisplay myDisplay = LedDisplay(dataPin, registerSelect, clockPin, enable, reset, displayLength); int brightness = 15; // screen brightness void setup() { // initialize the display library: myDisplay.begin(); // set the brightness of the display: myDisplay.setBrightness(brightness); } void loop() { // set the cursor to 0: myDisplay.home(); // print the millis: myDisplay.print("ms:"); myDisplay.print(millis()); }
Connections
5 signals need to connect from the display to pins on the teensy. It does not matter which pins you use, as long as you list them correctly when creating the LedDisplay object. 3 pins need to connect to ground and 4 connect to +5 volt power. The remaining two pins should be left unconnected.
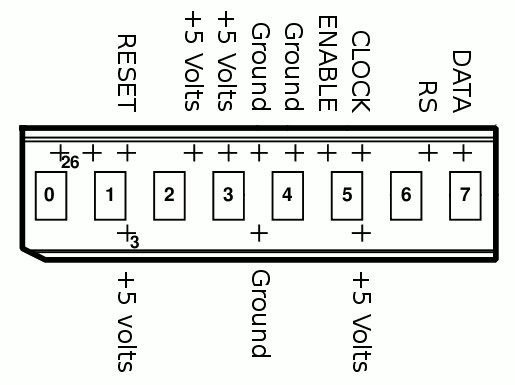